- Published on
Working with DND kit
(Please mindful, this is a draft, and I will be updating this quite often) DND Kit
This is part 1 of series of comparing different DND libraries. I started with exploring DND kit library. Goal is to create a simplest possible component with drag and drop feature.
I started with usual npx create-react-app draganddrop
then cd draganddrop && npm start
Sweet, i hope hello world CRA app is working for you.
Then, simply add DND kit by npm install @dnd-kit/core
Modify App.js to following
import logo from './logo.svg';
import './App.css';
import React, {useState} from 'react';
import {DndContext} from '@dnd-kit/core';
import {Droppable} from './Droppable';
import {Draggable} from './Draggable';
function App() {
const [isDropped, setIsDropped] = useState(false);
const draggableMarkup = (<Draggable><img src={logo} className="App-logo" alt="logo"/></Draggable>);
function handleDragEnd(event) {
if (event.over && event.over.id === 'droppable') {
setIsDropped(true);
}
}
return (<div className="App">
<header className="App-header">
<DndContext onDragEnd={handleDragEnd}>
{!isDropped ? draggableMarkup : null}
<p>This is a simple demo of DND Kit.</p>
<Droppable>
{isDropped ? draggableMarkup : 'Drop Logo here'}
</Droppable>
</DndContext>
</header>
</div>);
}
export default App;
Add draggable.js
import React from 'react';
import {useDraggable} from '@dnd-kit/core';
export function Draggable(props) {
const {attributes, listeners, setNodeRef, transform} = useDraggable({
id: 'draggable',
});
const style = transform ? {
transform: `translate3d(${transform.x}px, ${transform.y}px, 0)`,
} : undefined;
return (
<div ref={setNodeRef} style={style} {...listeners} {...attributes}>
{props.children}
</div>
);
}
Add Droppable.js
import React from 'react';
import {useDroppable} from '@dnd-kit/core';
export function Droppable(props) {
const {isOver, setNodeRef} = useDroppable({
id: 'droppable',
});
const style = {
color: isOver ? 'green' : undefined,
};
return (
<div ref={setNodeRef} style={style}>
{props.children}
</div>
);
}
Changed a little bit of css to this:
body{
background-color: #31333a;
}
.App {
text-align: center;
}
.App-logo {
height: 40vmin;
pointer-events: none;
}
@media (prefers-reduced-motion: no-preference) {
.App-logo {
animation: App-logo-spin infinite 20s linear;
}
}
.App-header {
min-height: 100vh;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
font-size: calc(10px + 1.5vmin);
color: white;
}
.App-link {
color: #61dafb;
}
@keyframes App-logo-spin {
from {
transform: rotate(0deg);
}
to {
transform: rotate(360deg);
}
}
And you should have your 1st working DND Kit component working
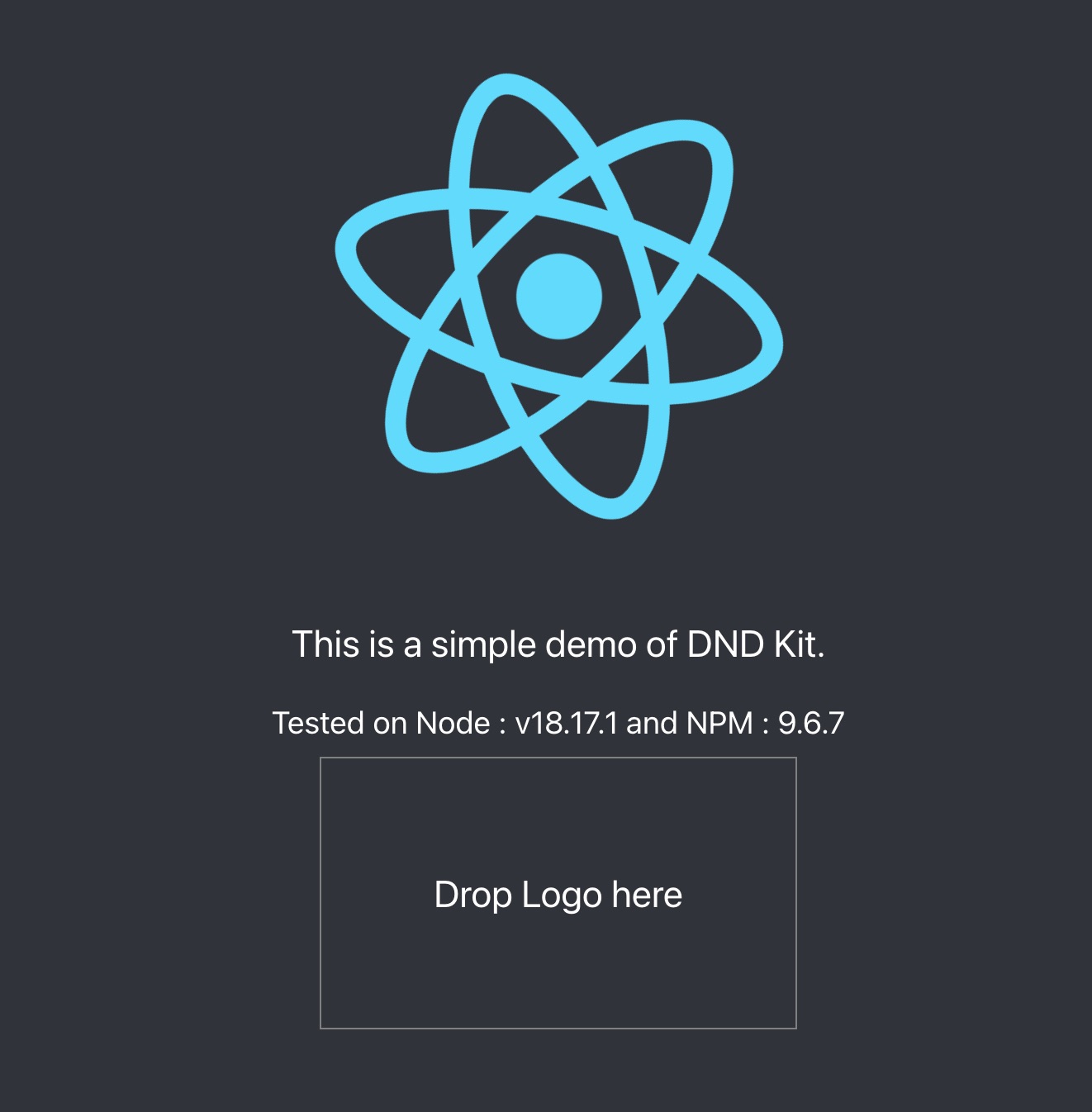
Discuss on Twitter • View on GitHub